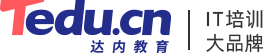
For investors
股價(jià):
5.36 美元 %For investors
股價(jià):
5.36 美元 %認(rèn)真做教育 專心促就業(yè)
參賽人:陳彬豪
獲得獎(jiǎng)項(xiàng):三等獎(jiǎng)
內(nèi)容:
第一步,就是先把單個(gè)格子的屬性創(chuàng)建出來(lái)
public class Cell { int row; int col; int color; static int indexRow = 10000;//這個(gè)參數(shù)是上下穿墻用的,為了保證不用完,要盡可能的大 static int indexCol = 10000;//這個(gè)參數(shù)是左右穿墻用的,為了保證不用完,要盡可能的大 public Cell(){//對(duì)于父類,無(wú)參數(shù)構(gòu)造器不一定用到,應(yīng)該習(xí)慣的寫(xiě)上 } public Cell(int x, int y, int color) { super(); this.row = x; this.col = y; this.color = color; } //各屬性的get set方法 public int getColor() { return color; } public void setColor(int color) { this.color = color; } public int getRow() { return row; } public void setRow(int x) { this.row = x; } public int getCol() { return col; } public void setCol(int y) { this.col = y; } /**向X軸負(fù)正向走*/ public void left(){ row = (indexRow+(--row))%40;/*這就是穿墻的關(guān)鍵,40是貪吃蛇墻的長(zhǎng),%40就能使蛇走不出墻,注意!!(--row)不能寫(xiě)成(row--) */ } /**向X軸負(fù)方向走*/ public void right(){ row= (indexRow+(++row))%40; } /**向Y軸負(fù)方向走*/ public void up(){ col = (indexCol+(--col))%40; } /**向Y軸正方向走*/ public void down(){ col = (indexCol+(++col))%40; } public String toString(){ return row+","+col; } }
第二步,對(duì)蛇身的構(gòu)造,以及蛇運(yùn)動(dòng)的方法
import java.util.Arrays; public class Body extends Cell{ /**蛇身的顏色*/ public static final int BADY_COLOR = 0x66ccff; /** 身體 */ public Cell[] bodys; /**頭*/ protected Cell head; public Body(){ bodys = new Cell[5]; head = new Cell(4,10,BADY_COLOR); bodys[0] = head; bodys[1] = new Cell(3,10,BADY_COLOR); bodys[2] = new Cell(2,10,BADY_COLOR); bodys[3] = new Cell(1,10,BADY_COLOR); bodys[4] = new Cell(0,10,BADY_COLOR); } /**絕對(duì)左移*/ public Cell[] moveLeft() { int i; worm Worm = new worm(); //當(dāng)前蛇身的長(zhǎng)度 int length = Worm.getBodyCellsLength(); //對(duì)蛇身數(shù)組的擴(kuò)容 bodys = Arrays.copyOf(bodys, length); for(i = length-1;i>0;i--){ if(bodys[i]==null){//如果吃了食物就會(huì)擴(kuò)容,擴(kuò)容的新元素為null bodys[i]=new Cell(); } bodys[i].col=bodys[i-1].col; bodys[i].row=bodys[i-1].row; } head.left(); return bodys; } /**絕對(duì)左移*/ public Cell[] moveRight() { int i; worm Worm = new worm(); int length = Worm.getBodyCellsLength(); bodys = Arrays.copyOf(bodys, length); for(i = length-1;i>0;i--){ if(bodys[i]==null){ bodys[i]=new Cell(); } bodys[i].setCol(bodys[i-1].col); bodys[i].setRow(bodys[i-1].row); } head.right(); return bodys; } /**絕對(duì)上移*/ public Cell[] goUp() { int i; worm Worm = new worm(); int length = Worm.getBodyCellsLength(); bodys = Arrays.copyOf(bodys, length); for(i = length-1;i>0;i--){ if(bodys[i]==null){ bodys[i]=new Cell(); } bodys[i].col=bodys[i-1].col; bodys[i].row=bodys[i-1].row; } head.up(); return bodys; } /**絕對(duì)下移*/ public Cell[] goDown() { int i; worm Worm = new worm(); int length = Worm.getBodyCellsLength(); bodys = Arrays.copyOf(bodys, length); for(i = length-1;i>0;i--){ if(bodys[i]==null){ bodys[i]=new Cell(); } bodys[i].col=bodys[i-1].col; bodys[i].row=bodys[i-1].row; } head.down(); return bodys; } public Cell[] getBody(){ return bodys; } public String toString(){ return Arrays.toString(bodys); } }
第三步 繪制動(dòng)作方法和繪圖
import java.awt.Color; import java.awt.Font; import java.awt.Graphics; import java.awt.Image; import java.awt.LayoutManager; import java.awt.event.KeyEvent; import java.awt.event.KeyListener; import java.awt.image.ImageObserver; import java.util.Arrays; import java.util.Random; import java.util.Timer; import java.util.TimerTask; import javax.swing.ImageIcon; import javax.swing.JButton; import javax.swing.JFrame; import javax.swing.JLabel; import javax.swing.JPanel; public class worm extends JPanel { public static final int ROWS = 40; public static final int COLS = 40; private Cell[][] wall = new Cell[ROWS][COLS]; /** 每一格的大小規(guī)格 */ public static final int CELL_SIZE = 10; /** 與邊框的距離 */ public static final int ADD = 20; public static final int BG_COLOR = 0xc3d5ea; /** 背景色 */ public static final int FONT_COLOR = 0xffffff; /** 邊框線條顏色 */ public static final int BORDER_COLOR = 0x667799; /** 食物的顏色 */ public static final int FOOD_COLOR = 0xff0000; /** 蛇身的顏色 */ public static final int BADY_COLOR = 0x66ccff; /** 是否吃到的判定 */ public static boolean eat = false; /** 正在向絕對(duì)上走 */ public static boolean goingUp = false; /** 正在向絕對(duì)下走 */ public static boolean goingDwon = false; /** 正在向絕對(duì)右走 */ public static boolean moveingRight = false; /** 正在向絕對(duì)左走 */ public static boolean moveingLeft = false; /**游戲結(jié)束*/ public static boolean gameOver = false; /**暫停判定*/ public static boolean pause = false; /**速度*/ public static int speed; /**分?jǐn)?shù)*/ public static int score; /** 食物 */ public static Cell food; /** 吃到食物前一格時(shí)的最后一格 */ public Cell tempBody; public Timer timer; public static Body body; /** 蛇身 */ public static Cell[] bodyCells; public static JFrame frame = new JFrame("貪吃蛇"); public static worm panel = new worm(null); // ------------------------------------開(kāi)始--------------------------------- public worm(LayoutManager layout){ super(layout); } public worm(){ } public static void main(String[] args) { Random ra = new Random(); /** 食物 */ food = new Cell(ra.nextInt(ROWS), ra.nextInt(COLS), FOOD_COLOR); frame.setSize((COLS +7) * CELL_SIZE-20, (ROWS+8) * CELL_SIZE + 90); frame.setResizable(false);//禁止窗口縮放 frame.setLocationRelativeTo(null);// 窗口居中//null是窗口的相對(duì)位置,沒(méi)有就是居中 frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);// 設(shè)置關(guān)閉窗口就關(guān)閉軟件 frame.add(panel);// 窗口中添加面板 frame.setVisible(true);// 顯示窗口 panel.action(); } /** 動(dòng)作流程 */ private void action() { startAction(); this.requestFocus();// 為當(dāng)前面板請(qǐng)求獲得輸入焦點(diǎn),this對(duì)象就獲得了輸入焦點(diǎn),以后任何的鍵盤(pán)輸入目標(biāo)就是這個(gè)面板 this.addKeyListener(new KeyListener() {// 添加鍵盤(pán)監(jiān)聽(tīng), public void keyPressed(KeyEvent e) {// 按鍵按下去 System.out.println("type:" + e.getKeyCode()); int key = e.getKeyCode(); if(gameOver!= true){ switch (key) { case 'A': if(goingUp||goingDwon){ moveLeftAction(); pause=false; } break; case 'D': if(goingUp || goingDwon){ moveRightAction(); pause=false; } break; case 'W': if(moveingRight || moveingLeft){ goUpAction(); pause=false; } break; case 'S': if(moveingLeft || moveingRight){ goDwonAction(); pause=false; } break; case KeyEvent.VK_SPACE: if(pause==false){ pauseAction(); pause=true; } } repaint(); } if(key==KeyEvent.VK_Q){ gameOver=true; pause=false; timer.cancel(); repaint(); } if(gameOver){ if(key==KeyEvent.VK_ENTER){ startAction(); } } /**ESC 退出系統(tǒng)*/ if(key==KeyEvent.VK_ESCAPE){ System.exit(0); } } public void keyReleased(KeyEvent e) { int key = e.getKeyCode(); }// 按鍵松開(kāi) public void keyTyped(KeyEvent e) { int key = e.getKeyCode(); if(key=='T'){ System.exit(0); } }// 按鍵按一下 }); } /**開(kāi)始流程*/ public void startAction(){ gameOver = false; pause = false; score = 0; level(); body = new Body(); bodyCells = body.bodys; newFood(); moveingRight = true; timer = new Timer(); timer.schedule(new TimerTask() { public void run() { TempBody(bodyCells); bodyCells = body.moveRight(); level(); gameOverAction(); bodyCells = grow(bodyCells, food, tempBody); repaint(); } }, 500, speed); repaint(); } /**結(jié)束流程*/ public void gameOverAction() { if (gameOver()) { System.out.println("gameOver"); timer.cancel(); } } private void pauseAction() { timer.cancel(); pause = true; } /** 向上走流程 */ private void goUpAction() { timer.cancel(); goingUp = true; goingDwon = false; moveingRight = false; moveingLeft = false; level(); timer = new Timer(); timer.schedule(new TimerTask() { public void run() { TempBody(bodyCells); bodyCells = body.goUp(); gameOverAction(); bodyCells = grow(bodyCells, food, tempBody); repaint(); } }, 0, speed); } /** 向下走流程 */ private void goDwonAction() { timer.cancel(); goingUp = false; goingDwon = true; moveingRight = false; moveingLeft = false; level(); timer = new Timer(); timer.schedule(new TimerTask() { public void run() { TempBody(bodyCells); bodyCells = body.goDown(); gameOverAction(); bodyCells = grow(bodyCells, food, tempBody); repaint(); } }, 0, speed); } /** 向左走流程 */ private void moveLeftAction() { timer.cancel(); goingUp = false; goingDwon = false; moveingRight = false; moveingLeft = true; level(); timer = new Timer(); timer.schedule(new TimerTask() { public void run() { TempBody(bodyCells); bodyCells = body.moveLeft(); gameOverAction(); bodyCells = grow(bodyCells, food, tempBody); repaint(); } }, 0, speed); } /** 想右走流程 */ private void moveRightAction() { timer.cancel(); goingUp = false; goingDwon = false; moveingRight = true; moveingLeft = false; level(); timer = new Timer(); timer.schedule(new TimerTask() { public void run() { TempBody(bodyCells); bodyCells = body.moveRight(); gameOverAction(); bodyCells = grow(bodyCells, food, tempBody); repaint(); } }, 0, speed); } /** 吃到食物前一格時(shí)的最后一格 */ public void TempBody(Cell[] bodyCells) { tempBody = new Cell(bodyCells[bodyCells.length - 1].row, bodyCells[bodyCells.length - 1].col, bodyCells[bodyCells.length - 1].color); } /** 增加1格 tempBody:吃到食物前一格時(shí)的最后一格 */ public Cell[] grow(Cell[] bodyCells, Cell food, Cell tempBody) { if (eatFood(bodyCells[0], food)) { bodyCells = Arrays.copyOf(bodyCells, bodyCells.length + 1); bodyCells[bodyCells.length - 1] = tempBody; score = (bodyCells.length-5)*10; newFood(); return bodyCells; } return bodyCells; } /** 判斷是否吃到食物 */ public static boolean eatFood(Cell head, Cell food) { eat = food.row == head.row && food.col == head.col; return eat; } /** 刷新食物 */ public void newFood() { if (eat) { Random ra = new Random(); /** 食物 */ food = new Cell(ra.nextInt(20), ra.nextInt(20), FOOD_COLOR); for(Cell cell: bodyCells){ if(food.row==cell.row && food.col== cell.col){ newFood(); } } eat = false; } } private void level(){ int index = 100; int scoreIndex = score; speed=200; if(scoreIndex>=index){ speed-=50; scoreIndex-=index; } if(scoreIndex>=index){ speed-=50; scoreIndex-=index; index=50; } if(scoreIndex>=index){ speed-=50; scoreIndex-=index; index=100; } if(scoreIndex>=index){ speed-=10; scoreIndex-=index; } } /** 游戲結(jié)束判定 */ private boolean gameOver() { for (int i = 1; i < bodyCells.length; i++) { gameOver = bodyCells[0].row == bodyCells[i].row && bodyCells[0].col == bodyCells[i].col; if (gameOver == true) { return gameOver; } } return gameOver; } public int getBodyCellsLength() { return bodyCells.length; } public worm getworm(){ worm we = new worm(); return we; } // ------------------------------------------------------------------------------------------- /** JPanel 類利用paint(圖畫(huà))方法繪制界面,子類重寫(xiě)paint方法可以修改繪圖邏輯 */ public void paint(Graphics g) { paintBackBorder(g); //paintWall(g);//繪制墻的格子,方便蛇的運(yùn)動(dòng),但玩的時(shí)候最好注釋掉,這要更美觀 paintBody(g); paintFood(g); paintScore(g); paintTetrisBorder(g); } /** 填充背景 */ private void paintBackBorder(Graphics g) { g.setColor(new Color(0x000000)); g.fillRect(ADD, ADD+CELL_SIZE * 10, CELL_SIZE * ROWS, CELL_SIZE * COLS); g.setColor(new Color(0xc3d5ea)); g.fillRect(ADD, ADD, CELL_SIZE *ROWS, CELL_SIZE * 10); } /** 繪制墻 */ private void paintWall(Graphics g) { for (int row = 0; row < ROWS; row++) { for (int col = 0; col < COLS; col++) { Cell cell = wall[row][col]; // 墻的格子 // g.setColor(new Color(BORDER_COLOR)); // g.drawRect(ADD +col * CELL_SIZE, ADD+10*CELL_SIZE+row * CELL_SIZE, // CELL_SIZE, CELL_SIZE); } } } /** 繪制食物 */ private void paintFood(Graphics g) { int row = food.getRow(); int col = food.getCol(); g.setColor(new Color(food.getColor())); g.fill3DRect(ADD + row * CELL_SIZE, ADD + col * CELL_SIZE+CELL_SIZE * 10, CELL_SIZE, CELL_SIZE, true); g.setColor(new Color(0xffffff)); g.draw3DRect(ADD + row * CELL_SIZE, ADD + col * CELL_SIZE+CELL_SIZE * 10, CELL_SIZE, CELL_SIZE, true); } /** 繪制蛇身 */ private void paintBody(Graphics g) { for (Cell cell : bodyCells) { int row = cell.getRow(); int col = cell.getCol(); g.setColor(new Color(0x66ccff)); g.fill3DRect(ADD + row * CELL_SIZE, ADD + col * CELL_SIZE+CELL_SIZE * 10, CELL_SIZE, CELL_SIZE, true); g.setColor(new Color(0xffffff)); g.draw3DRect(ADD + row * CELL_SIZE, ADD + col * CELL_SIZE+CELL_SIZE * 10, CELL_SIZE, CELL_SIZE, true); } } /** 繪制文字 */ private void paintScore(Graphics g) { Font font = new Font(getFont().getName(), Font.BOLD, 35); String str = "SCORE : " + score; g.setColor(new Color(FONT_COLOR)); g.setFont(font); g.drawString(str, 13 * CELL_SIZE, 7 * CELL_SIZE); str = "[SPACE]PAUSE"; font = new Font(getFont().getName(), Font.BOLD, 20); g.setFont(font); g.drawString(str, 4 * CELL_SIZE, 11 * CELL_SIZE); str = "[Q]QUIT"; g.drawString(str, 25 * CELL_SIZE, 11 * CELL_SIZE); if(pause){ font = new Font(getFont().getName(), Font.BOLD, 100); g.setFont(font); str = "PAUSE"; g.drawString(str, 6 * CELL_SIZE, 35 * CELL_SIZE); } if(gameOver){ font = new Font(getFont().getName(), Font.BOLD, 60); g.setFont(font); str = "GAME OVER "; g.drawString(str, 4 * CELL_SIZE, 25 * CELL_SIZE); font = new Font(getFont().getName(), Font.BOLD, 20); g.setFont(font); str = "YOUR SCORE "; g.drawString(str, 16 * CELL_SIZE, 30 * CELL_SIZE); font = new Font(getFont().getName(), Font.BOLD, 100); g.setFont(font); str = scoreString(score); g.drawString(str, 6 * CELL_SIZE, 40 * CELL_SIZE); str = "[ENTER] START "; font = new Font(getFont().getName(), Font.BOLD, 40); g.setFont(font); g.drawString(str, 7 * CELL_SIZE, 45* CELL_SIZE); } } /** 繪制邊框 */ private void paintTetrisBorder(Graphics g) { g.setColor(new Color(BORDER_COLOR));// 繪制邊線 g.drawRect(ADD, ADD+CELL_SIZE * 10, CELL_SIZE * COLS, CELL_SIZE * ROWS); g.drawRect(ADD, ADD, CELL_SIZE*COLS, CELL_SIZE* 10); } private String scoreString(int score){ String str= ""+score; for(int i=0;i<3-(str.length())/2;i++){ if(str.length()%2==0){ str+=" "; } if(str.length()%2!=0){ str=" "+str; } } return str; } }
以上就是完整的代碼,親測(cè)能用,復(fù)制粘貼就用運(yùn)行,基本上都有注釋,看注釋就能明白了,寫(xiě)程序加注釋是很好的習(xí)慣,不然不只是用的人不知道說(shuō)什么,寫(xiě)到后面很可能連自己都不知哪個(gè)方法是干什么的!!!
寫(xiě)這篇東西純屬學(xué)習(xí)上的交流,給多少分就隨意把,本人覺(jué)得征文的這個(gè)系統(tǒng)太坑爹了,進(jìn)來(lái)了居然不打分不給走(x.x)
【免責(zé)聲明】本文部分系轉(zhuǎn)載,轉(zhuǎn)載目的在于傳遞更多信息,并不代表本網(wǎng)贊同其觀點(diǎn)和對(duì)其真實(shí)性負(fù)責(zé)。如涉及作品內(nèi)容、版權(quán)和其它問(wèn)題,請(qǐng)?jiān)?0日內(nèi)與聯(lián)系我們,我們會(huì)予以更改或刪除相關(guān)文章,以保證您的權(quán)益!